Unveiling 2-Edge Connected Components with DFS Lowlinking
Written on November 25, 2024
Views : Loading...
Unveiling 2-Edge Connected Components with DFS Lowlinking
Category: Computer Science
Tags: Graph Theory, Algorithms, Depth-First Search, Bridges, Connectivity, Data Structures
Introduction
In the realm of graph theory, understanding the connectivity of a network is crucial for numerous applications, from network reliability to circuit design. One fascinating aspect of connectivity is identifying 2-edge connected components in an undirected graph. These components remain connected even after the removal of any single edge, highlighting their robustness against single points of failure.
In this blog post, we'll delve into an efficient algorithm using Depth-First Search (DFS) and the concept of low-link values to find and print all 2-edge connected components in an undirected graph. We'll break down the intuition, provide mathematical proofs, and walk through the algorithm step by step, ensuring even beginners can grasp the concepts.
Understanding Key Concepts
Undirected Graphs
An undirected graph is a set of objects (called vertices or nodes) connected by edges, where the edges have no direction. Formally, a graph is represented as $G = (V, E)$, where $V$ is the set of vertices and $E$ is the set of edges.
Connectivity
A graph is connected if there's a path between every pair of vertices. 2-edge connected components are maximal subsets of vertices where any two vertices are connected by at least two disjoint paths, meaning the removal of any single edge does not disconnect the component.
Bridges
An edge is called a bridge if its removal increases the number of connected components in the graph. Identifying bridges is key to finding 2-edge connected components because bridges are the edges that, when removed, split the graph into separate components.
Depth-First Search (DFS)
DFS is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It's particularly useful for connectivity problems in graphs.
Intuition Behind the Algorithm
The core idea is to use DFS to traverse the graph and compute two values for each node:
- In-Time ($in_time[u]$): The time when a node $u$ is first visited during the DFS traversal.
- Low-Link Value ($low[u]$): The lowest in-time reachable from $u$ (including $u$ itself and its descendants in the DFS tree).
By comparing the in-time and low-link values, we can identify bridges:
- If for an edge $(u, v)$, $low[v] > in_time[u]$, then $(u, v)$ is a bridge.
Once we've found all the bridges, we can perform another DFS, this time avoiding the bridges, to identify and count the 2-edge connected components.
Detailed Explanation
Finding Bridges Using DFS Lowlinking
Step 1: Initialize Variables
We initialize:
- Timer: A global variable to assign in-time values.
- In-Time Array ($in_time$): Stores the time at which each node is visited.
- Low-Link Array ($low$): Stores the lowest in-time reachable from each node.
- Bridges Set: To store all identified bridges.
Step 2: DFS Traversal
We perform DFS starting from an arbitrary node. For each node $u$, we:
- Set $in_time[u] = low[u] = timer++$.
- For each adjacent node $v$:
- If $v$ is the parent of $u$, we skip it to prevent trivial cycles.
- If $v$ is not visited, we recursively call DFS for $v$.
- After returning, we update $low[u] = \min(low[u], low[v])$.
- Bridge Condition: If $low[v] > in_time[u]$, then $(u, v)$ is a bridge.
- If $v$ is already visited (back edge), we update $low[u] = \min(low[u], in_time[v])$.
Mathematical Proof
The crucial part is proving that an edge $(u, v)$ is a bridge if and only if $low[v] > in_time[u]$.
- If $low[v] > in_time[u]$: There is no back edge from the subtree rooted at $v$ to $u$ or any of its ancestors. Removing $(u, v)$ disconnects $v$'s subtree from $u$, making $(u, v)$ a bridge.
- If $low[v] \leq in_time[u]$: There is a back edge, so even if we remove $(u, v)$, there is another path connecting $v$ and $u$, so $(u, v)$ is not a bridge.
Code Snippet
void dfs(int u, int parent) {
in_time[u] = low[u] = timer++;
for(int v : graph[u]) {
if(v == parent) continue;
if(!in_time[v]) {
dfs(v, u);
low[u] = min(low[u], low[v]);
if(low[v] > in_time[u]) {
bridges.insert({u, v});
bridges.insert({v, u});
}
} else {
low[u] = min(low[u], in_time[v]);
}
}
}
Finding 2-Edge Connected Components
Step 3: Second DFS to Identify Components
After identifying all bridges, we perform another DFS to find 2-edge connected components by:
- Initializing a visited array to keep track of visited nodes.
- For each unvisited node, we perform DFS, assigning a component ID to all reachable nodes without crossing any bridges.
- We skip any edge that's a bridge during this traversal.
Code Snippet
void dfs_component(int u, int component_id) {
visited[u] = component_id;
for(int v : graph[u]) {
if(bridges.count({u, v})) continue;
if(!visited[v]) {
dfs_component(v, component_id);
}
}
}
Explanation of the Algorithm
- Why Skip Bridges? Bridges are the edges that, if removed, increase the number of connected components. By skipping them, we ensure that we only traverse within a 2-edge connected component.
- Component Identification: Each DFS traversal without crossing a bridge will visit all nodes in a single 2-edge connected component.
Complete Algorithm Implementation
Here's the complete code combining both steps:
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
vector<int> graph[100005];
vector<int> in_time(100005, 0);
vector<int> low(100005, 0);
set<pair<int, int>> bridges;
vector<int> visited(100005, 0);
int timer = 1;
void dfs(int u, int parent) {
in_time[u] = low[u] = timer++;
for(int v : graph[u]) {
if(v == parent) continue;
if(!in_time[v]) {
dfs(v, u);
low[u] = min(low[u], low[v]);
if(low[v] > in_time[u]) {
bridges.insert({u, v});
bridges.insert({v, u});
}
} else {
low[u] = min(low[u], in_time[v]);
}
}
}
void dfs_component(int u, int component_id) {
visited[u] = component_id;
for(int v : graph[u]) {
if(bridges.count({u, v})) continue;
if(!visited[v]) {
dfs_component(v, component_id);
}
}
}
int main() {
int n, m;
cin >> n >> m;
// Build the graph
for(int i = 0; i < m; i++) {
int u, v;
cin >> u >> v;
graph[u].push_back(v);
graph[v].push_back(u);
}
// Find bridges
for(int i = 1; i <= n; i++) {
if(!in_time[i]) dfs(i, -1);
}
// Find 2-edge connected components
int component_id = 1;
map<int, vector<int>> components;
for(int i = 1; i <= n; i++) {
if(!visited[i]) {
dfs_component(i, component_id);
component_id++;
}
}
// Output the components
cout << component_id - 1 << endl;
for(int i = 1; i < component_id; i++) {
cout << components[i].size() << " ";
for(int node : components[i]) {
cout << node << " ";
}
cout << endl;
}
return 0;
}
Explanation of the Code
- Graph Construction: Reads the number of nodes and edges and constructs the adjacency list.
- Finding Bridges: Calls
dfs
for each unvisited node to find all bridges. - Identifying Components: Uses
dfs_component
to assign component IDs, skipping bridges. - Output: Prints the number of 2-edge connected components and lists their nodes.
Conclusion
By leveraging DFS and low-link values, we can efficiently identify bridges in an undirected graph, which in turn allows us to find all 2-edge connected components. This algorithm is vital for understanding the resilience of networks and can be applied in various fields like network design, biology, and sociology.
Understanding this algorithm enhances our grasp of graph connectivity and traversal techniques. Future developments might focus on optimizing the algorithm for larger graphs or adapting it to dynamic graphs where edges are frequently added or removed.
References
Share this blog
Related Posts
24-11-2024
Dive deep into the Z Algorithm, a fundamental technique in string processing. This blog elucidates t...
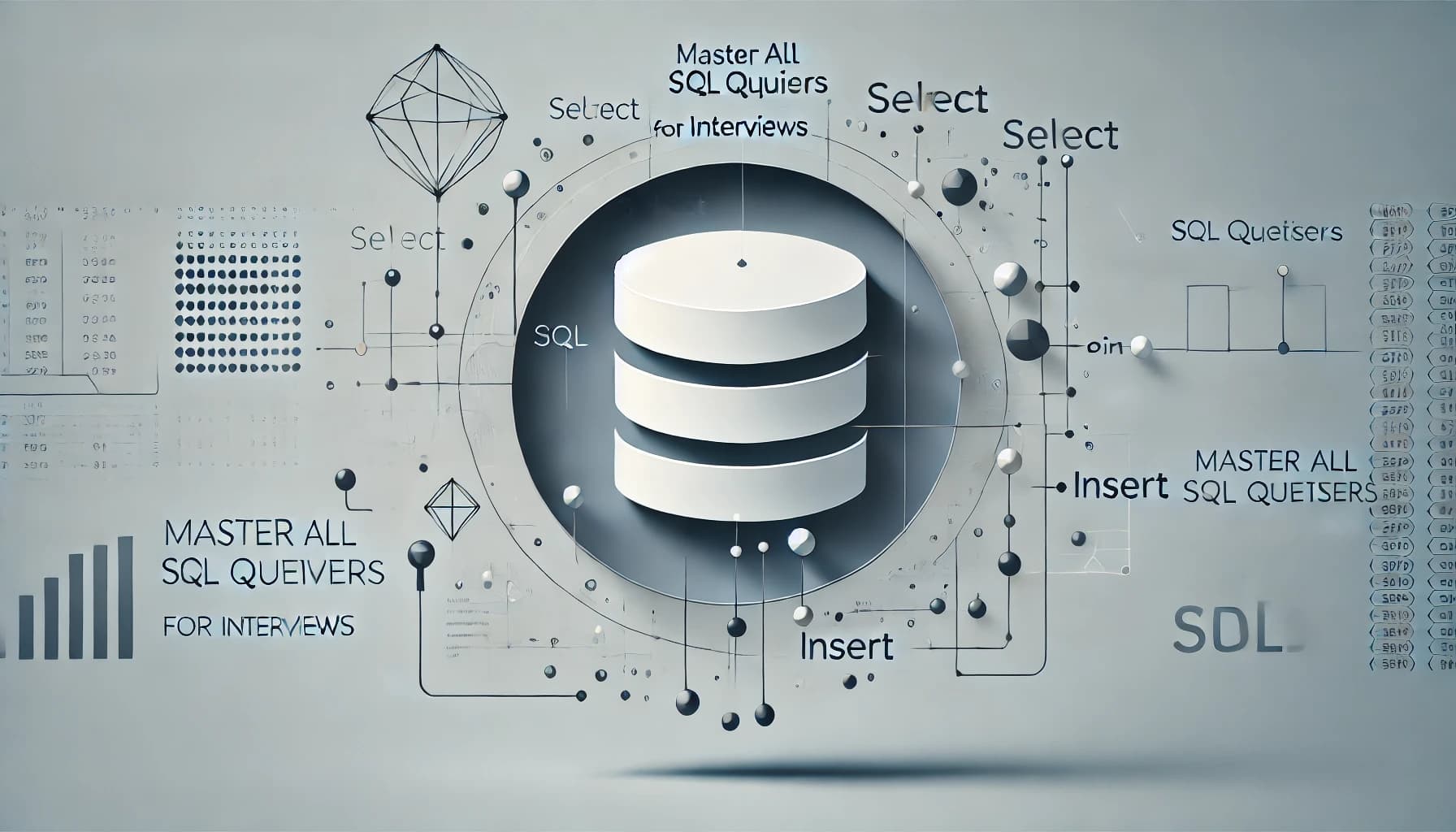
28-07-2024
In this comprehensive guide, you'll master essential SQL queries to ace your database-related interv...
04-11-2023
Explore the most efficient Sorting Algorithms for Competitive Programming and Interviews. Learn abou...
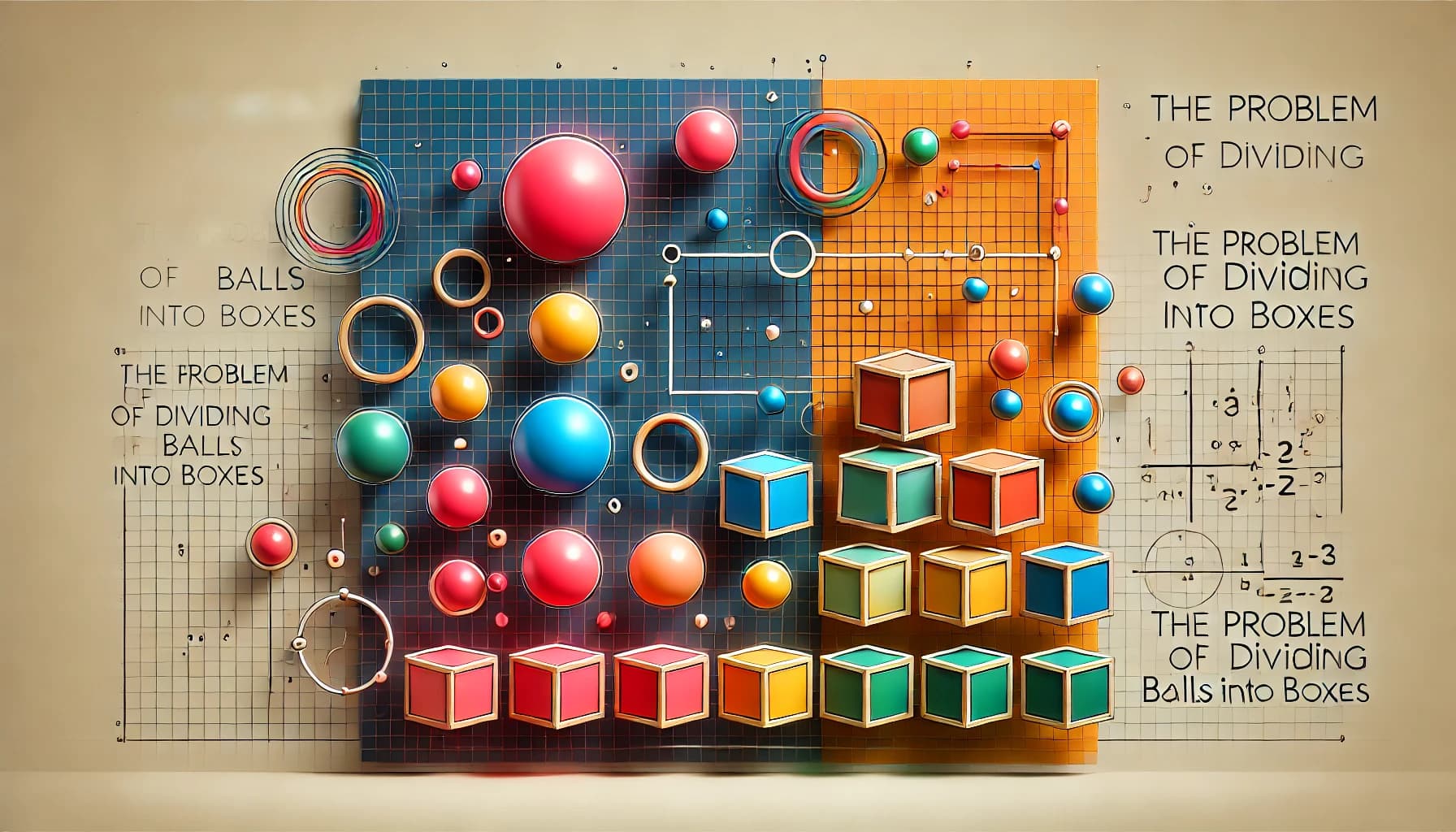
23-07-2023
This document discusses various variants of the problem of dividing n balls into *m* boxes. Markdown...
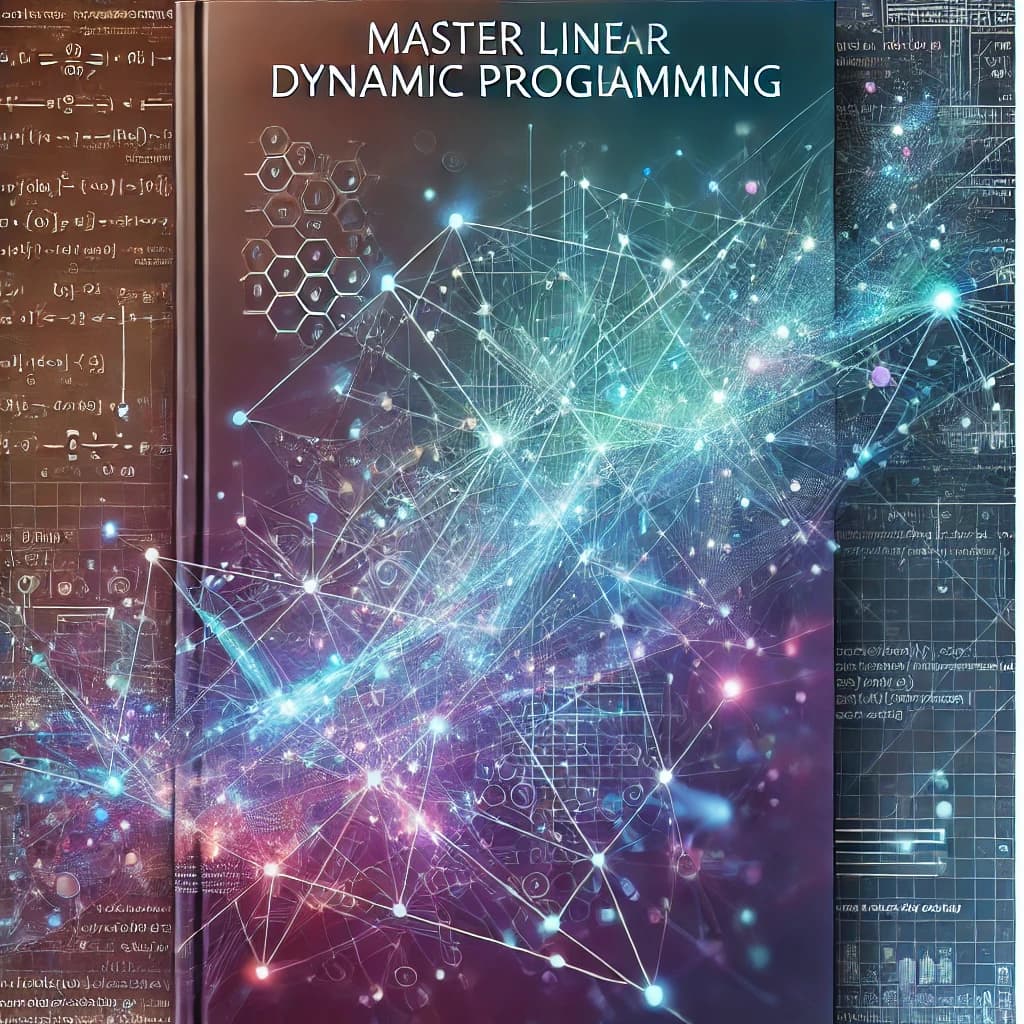
22-07-2023
Enhance your dynamic programming skills with our comprehensive collection of Linear DP problems from...
19-07-2023
Become a Tree Algorithms expert with our comprehensive solutions for CSES and various problem sets. ...
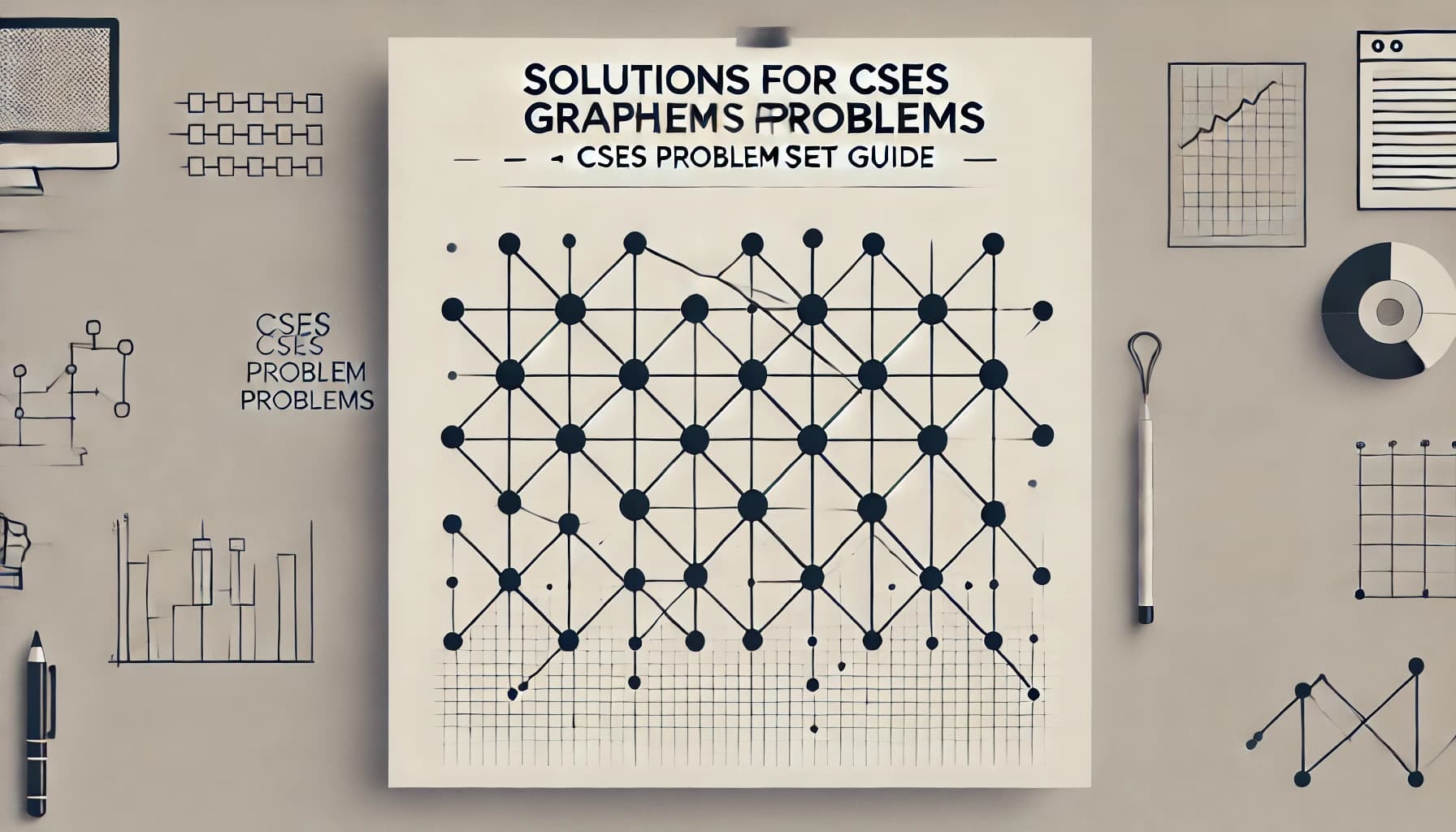
17-07-2023
Explore efficient solutions and master essential algorithms for CSES Graph problems in the CSES Prob...
15-07-2023
Explore efficient solutions to the Sorting and Searching section in the CSES Problem Set. Master ess...
12-07-2023
Explore efficient solutions to dynamic programming problems from the CSES problem set and other repu...