Mastering JavaScript Iterations: forEach, map, filter, reduce, find, and the Classic for Loop
Written on November 30, 2024
Views : Loading...
Introduction
Iteration is at the heart of programming. Whether you're traversing an array, manipulating data, or performing repetitive tasks, understanding how to efficiently loop through data structures is crucial. JavaScript offers a plethora of iteration methods, including the traditional for
loop and modern array methods like forEach
, map
, filter
, reduce
, and find
. Each has its unique features and use-cases, and choosing the right one can significantly enhance code readability and performance.
However, with great power comes great responsibility. Asynchronous programming introduces complexity, especially when combined with these iteration methods. Developers must be cautious to avoid common pitfalls that can lead to unexpected behavior or performance issues. In this blog post, we'll explore the differences between these iteration methods, delve into asynchronous programming concerns, and highlight best practices to ensure your code is both efficient and maintainable.
The Classic for
Loop
The for
loop is one of the most fundamental constructs in JavaScript, offering complete control over the iteration process.
Definition and Syntax
The for
loop repeats a block of code a certain number of times, based on a condition. Its syntax is:
for (initialization; condition; increment) {
// Code to execute
}
- Initialization: Sets up a variable for tracking the loop's progress.
- Condition: Determines whether the loop should continue running.
- Increment: Updates the loop variable after each iteration.
Example
Let's iterate over an array of numbers and print each one:
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
In this example:
- We initialize
i
to0
. - The loop runs as long as
i
is less thannumbers.length
. - After each iteration,
i
increments by1
.
Use Cases
- When you need full control over the iteration process.
- When you need to manipulate the index directly.
- When performance is critical, and you want to avoid the overhead of higher-order functions.
The forEach
Method
Definition
forEach
is an array method that executes a provided function once for each array element.
Syntax
array.forEach(function(currentValue, index, array) {
// Code to execute
});
- currentValue: The current element being processed.
- index (optional): The index of the current element.
- array (optional): The array
forEach
was called upon.
Example
Printing each number in an array:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
Use Cases
- When you need to execute a function for each element without returning a new array.
- Improves code readability by abstracting the loop control variables.
Things to Note
forEach
cannot be stopped or broken before all elements have been iterated.- It doesn't return a value.
The map
Method
Definition
map
creates a new array populated with the results of calling a provided function on every element.
Syntax
let newArray = array.map(function(currentValue, index, array) {
// Return the new value
});
Example
Doubling each number in an array:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(function(number) {
return number * 2;
});
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Use Cases
- When you need to transform each element in an array.
- It returns a new array, leaving the original array unchanged.
The filter
Method
Definition
filter
creates a new array with all elements that pass the test implemented by the provided function.
Syntax
let filteredArray = array.filter(function(currentValue, index, array) {
// Return true to keep the element, false otherwise
});
Example
Filtering out even numbers:
const numbers = [1, 2, 3, 4, 5];
const oddNumbers = numbers.filter(function(number) {
return number % 2 !== 0;
});
console.log(oddNumbers); // Output: [1, 3, 5]
Use Cases
- When you need a subset of elements that meet certain criteria.
- Non-matching elements are omitted from the new array.
The reduce
Method
Definition
reduce
executes a reducer function on each element, resulting in a single output value.
Syntax
let result = array.reduce(function(accumulator, currentValue, index, array) {
// Return the updated accumulator
}, initialValue);
- accumulator: Accumulates the callback's return values.
- currentValue: The current element being processed.
- initialValue (optional): A value to use as the first argument to the first call of the callback.
Example
Summing all numbers in an array:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce(function(total, number) {
return total + number;
}, 0);
console.log(sum); // Output: 15
Use Cases
- Calculating a single value from an array (e.g., sum, product, average).
- Can also be used to flatten arrays or construct objects.
The find
Method
Definition
find
returns the value of the first element that satisfies the provided testing function.
Syntax
let foundElement = array.find(function(currentValue, index, array) {
// Return true when the element is found
});
Example
Finding the first number greater than 3
:
const numbers = [1, 2, 3, 4, 5];
const found = numbers.find(function(number) {
return number > 3;
});
console.log(found); // Output: 4
Use Cases
- When you need to retrieve a single element based on a condition.
- Stops execution once the element is found, improving performance.
Comparing Iteration Methods
When to Use What
for
Loop: Full control over the iteration, useful when you need to break out of a loop or manipulate the index.forEach
: Simple iterations where you don't need to modify the array or return a value.map
: When you need to transform every element and obtain a new array.filter
: Extracting elements that meet specific criteria into a new array.reduce
: Condensing an array into a single value (e.g., sum, product).find
: Quickly locating a single element in an array.
Performance Considerations
- Traditional Loops vs. Array Methods: Traditional loops can be faster due to less overhead but can be more verbose.
- Readability: Higher-order functions (
forEach
,map
, etc.) can make code more readable and expressive.
Asynchronous Programming Considerations
Asynchronous programming introduces challenges, especially when combined with iteration methods.
The Problem with Asynchronous Loops
Methods like forEach
, map
, and filter
do not handle asynchronous callbacks as you might expect. They don't wait for promises to resolve before moving to the next iteration.
Example of Unexpected Behavior
const urls = ['url1', 'url2', 'url3'];
urls.forEach(function(url) {
fetch(url).then(function(response) {
console.log(response);
});
});
console.log('All fetch calls initiated.');
You might expect that the console logs the responses before printing 'All fetch calls initiated.' However, the fetch calls are asynchronous, and the loop doesn't wait for them to complete.
Correcting the Issue with Async/Await
Using for...of
with async/await
(async function() {
const urls = ['url1', 'url2', 'url3'];
for (const url of urls) {
const response = await fetch(url);
console.log(response);
}
console.log('All fetch calls completed.');
})();
Using Promise.all
For parallel execution:
const urls = ['url1', 'url2', 'url3'];
const fetchPromises = urls.map(function(url) {
return fetch(url);
});
Promise.all(fetchPromises).then(function(responses) {
responses.forEach(function(response) {
console.log(response);
});
console.log('All fetch calls completed.');
});
Things to Be Careful About
- Avoid
await
inforEach
:forEach
doesn't handle promises well because it doesn't await the completion of asynchronous operations. - Consider Execution Order: Decide whether you need serial (one after another) or parallel execution.
- Error Handling: Use try-catch blocks or
.catch()
methods to handle errors in asynchronous code.
Best Practices While Coding
Keep Functions Pure
- Avoid side effects.
- Pure functions make testing and debugging easier.
Use Descriptive Variable Names
- Improves code readability.
- Makes maintenance easier for you and others.
Avoid Mutating Data
- Methods like
map
,filter
, andreduce
return new arrays. - Mutating the original data can lead to bugs that are hard to trace.
Handle Errors Gracefully
- Always anticipate possible errors, especially in asynchronous code.
- Use proper error handling mechanisms like try-catch blocks.
Optimize Performance
- Be mindful of time complexity, especially in nested loops.
- Use appropriate data structures and algorithms.
Write Modular Code
- Break down complex functions into smaller, reusable pieces.
- Enhances readability and maintainability.
Conclusion
Mastering JavaScript's iteration methods empowers you to write cleaner, more efficient code. Understanding when and how to use forEach
, map
, filter
, reduce
, find
, and the classic for
loop can significantly improve your programming skills. Additionally, being cautious with asynchronous operations within loops is essential to prevent unexpected behavior and performance issues.
By following best practices and being mindful of the intricacies of each method, you can write robust code that is both performant and easy to understand. As JavaScript continues to evolve, staying informed about these fundamental concepts will serve as a solid foundation for tackling more advanced topics.
Share this blog
Related Posts
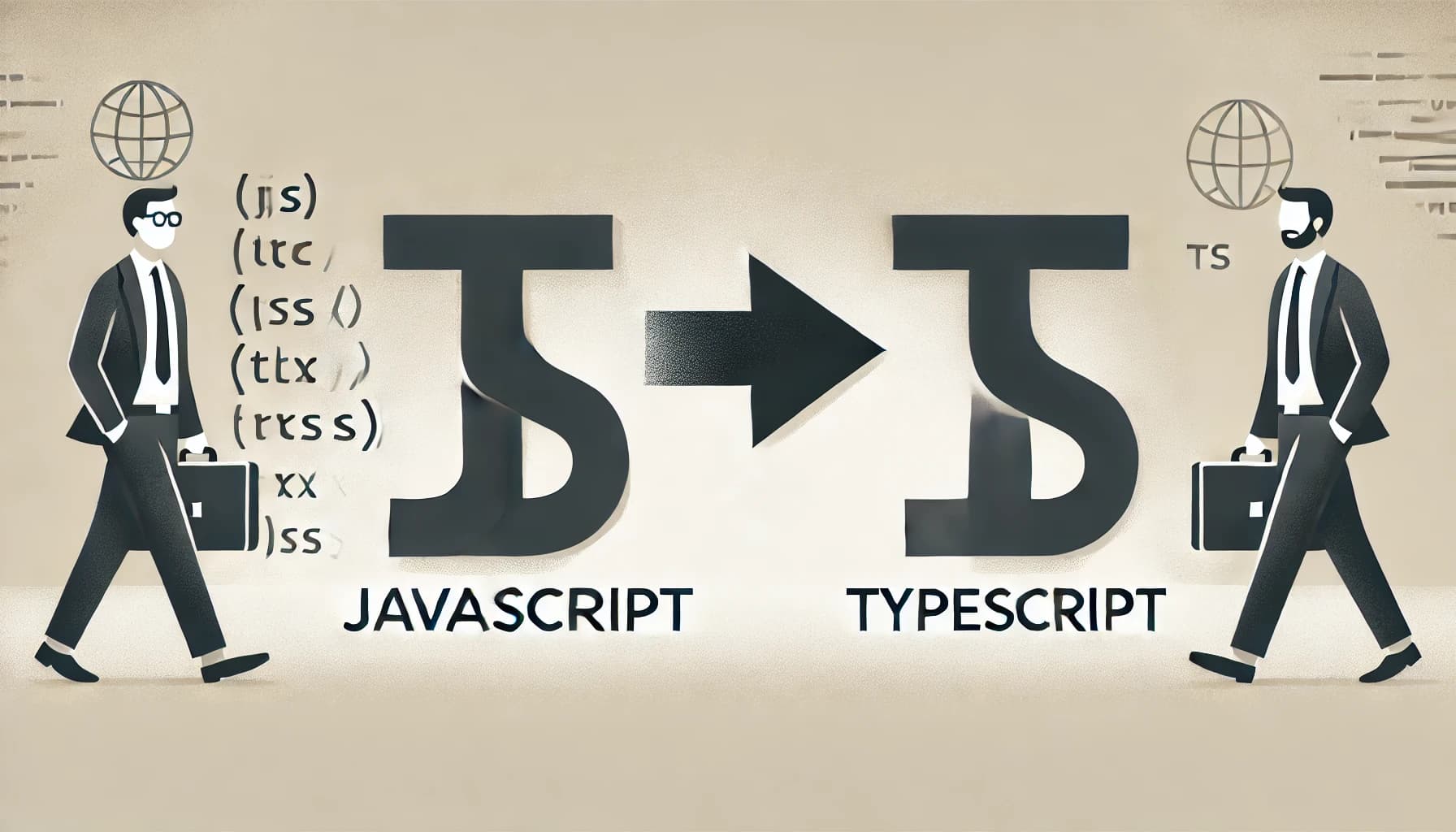
09-06-2023
Learn how to smoothly transition from JavaScript to TypeScript and unlock the benefits of static typ...
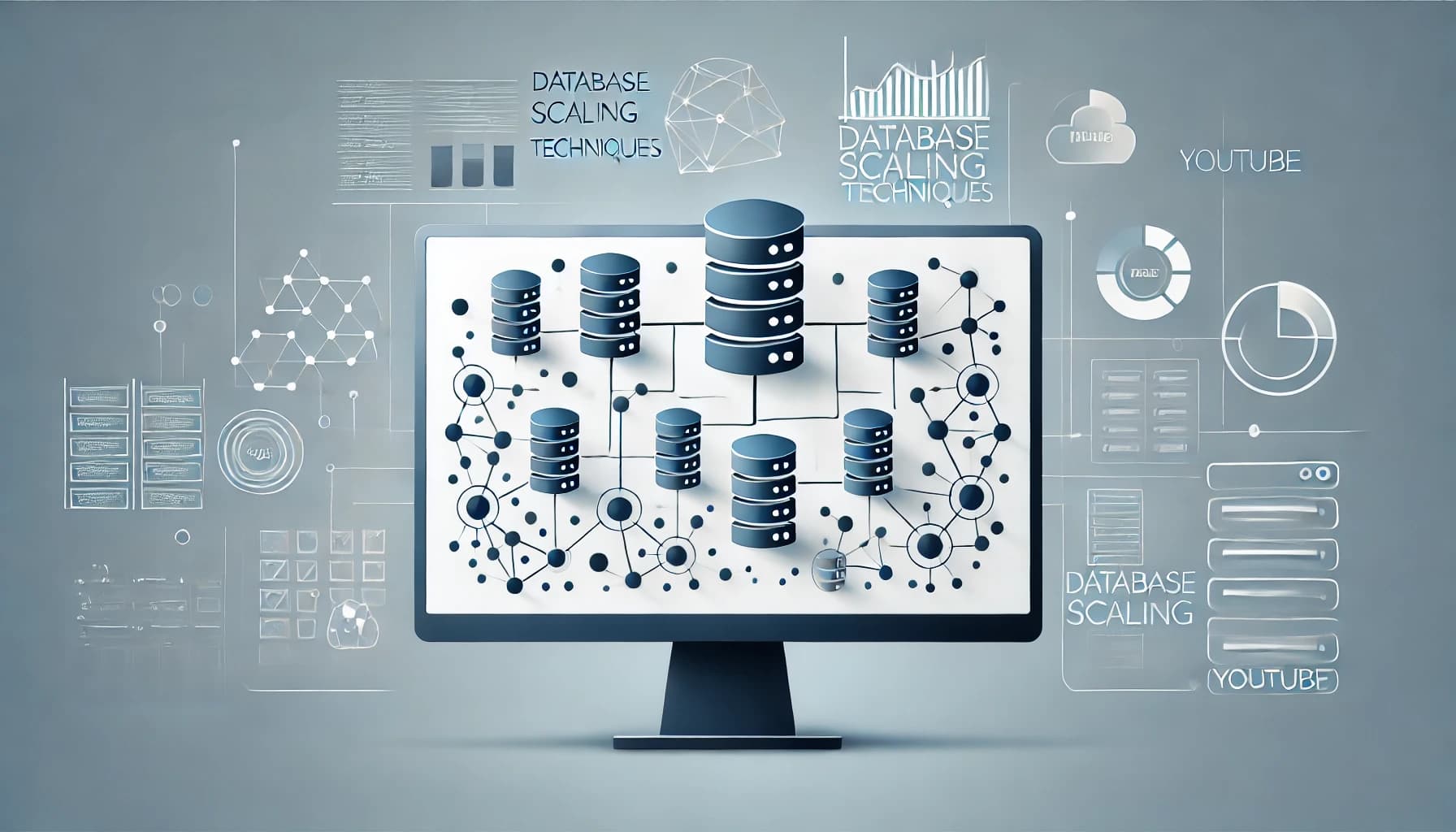
24-05-2023
Learn essential strategies for scaling your web application's database to accommodate a growing user...
18-04-2025
Explore effective strategies for enhancing the performance of serverless AI deployments on AWS Lambd...
17-04-2025
Explore how implementing DeepSeek's Distributed File System can significantly improve performance me...
16-04-2025
Explore how to implement scalable ML models using Kubernetes, focusing on metric improvements for de...
15-04-2025
Explore how microservices architecture can be enhanced with AI to improve performance and scalabilit...
14-04-2025
Explore how to implement real-time audio generation using Diffusion Transformer models with AudioX, ...
12-04-2025
Learn how to effectively deploy AI models at scale using Kubernetes and serverless architectures.
11-04-2025
Discover advanced techniques to optimize algorithms for real-time data streams and improve throughpu...